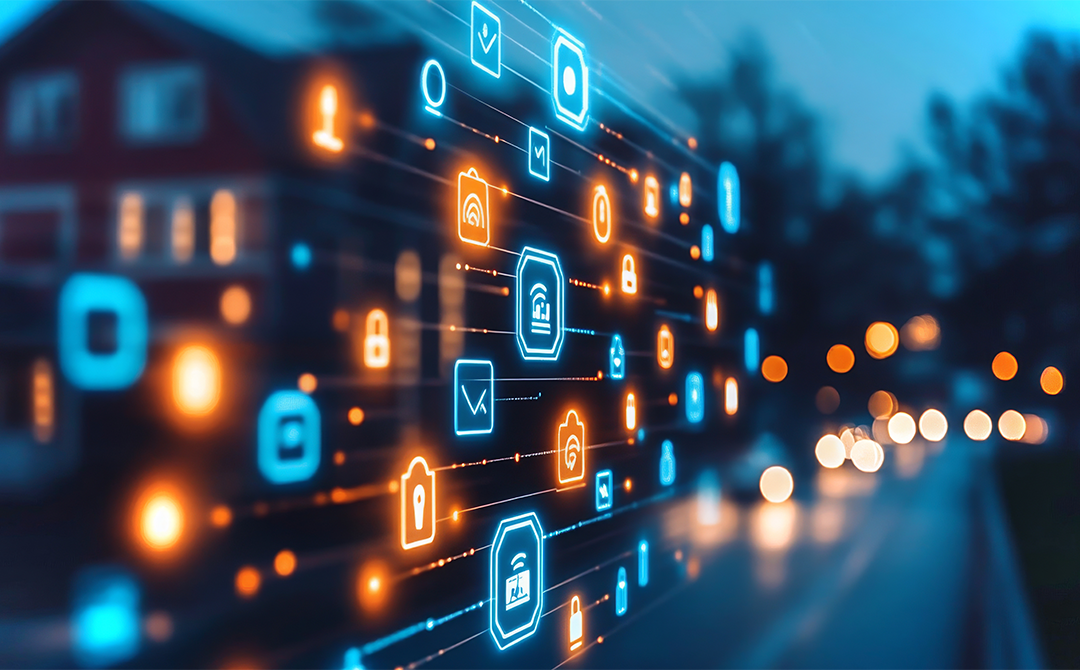
Introduction: What Are Databricks Apps?
Databricks Apps are a powerful new capability within the Databricks Lakehouse Platform that lets users build full-stack, interactive applications - right inside their Databricks workspace. Unlike external dashboarding or visualization tools like Streamlit, Flask, or BI software, Databricks Apps bring the UI layer into your workspace environment, leveraging the same governance and security you already use with Unity Catalog.
Think of them as embedded apps that can interact natively with Delta tables, ML models, and notebooks - making it easier to serve insights directly to business users and operational teams.
But why is this such a big deal?
In many data organizations today, teams struggle with what's often called the “last-mile problem.” Analysts and engineers spend weeks building data pipelines, transforming raw data into usable formats, and storing it in governed tables. But when it comes to actually delivering this data to business stakeholders in an interactive way - things fall apart.
- Tools like Power BI and Tableau are powerful but often require refresh schedules, connectors, and security configurations.
- Streamlit or Flask apps may require external hosting, separate CI/CD pipelines, and additional governance.
- Many business teams still export to Excel because that's the only accessible format available.
The “Last-Mile” Problem in Analytics
Despite our best pipelines and pristine Delta tables, this is often the real experience:
- The issue? Data is ready, but insights are delayed.
- The gap? We don't have native, interactive, business-friendly apps within our workspace.
Databricks Apps aim to solve this problem by enabling you to build UI directly on top of your Lakehouse assets - with no context switching. That means lower latency, simplified architecture, and faster insights delivery.
The Business Problems Databricks Apps Solve
Let's break it down:
What are Databricks Apps Today?
Databricks Apps are a transformative feature within the Databricks ecosystem, enabling developers to build, deploy, and share secure data and AI applications directly on the Databricks platform. This integration simplifies the development process, allowing for rapid creation of interactive, production-ready applications without the need for complex infrastructure management. (Source: Databricks)
Key Features and Capabilities
- Native Development Environment: Developers can create applications using popular Python frameworks such as Dash, Streamlit, Gradio, Flask, and Shiny. This flexibility allows for rapid development and iteration of data-driven applications. (Source: Databricks)
- Serverless Deployment: Databricks Apps run on automatically provisioned serverless compute resources, removing the overhead of managing infrastructure and enabling seamless scaling.
- Integrated Governance and Security: With built-in support for Unity Catalog, OAuth 2.0, and single sign-on (SSO), Databricks Apps ensure that applications adhere to enterprise-grade security and governance standards. (Source: Databricks Docs)
- Simplified Sharing and Collaboration: Applications can be easily shared with users within the organization, promoting collaboration and enabling non-technical stakeholders to interact with data insights through intuitive interfaces. (Source: Databricks Docs)
Transforming Data Interaction
By leveraging Databricks Apps, organizations can transform the way they interact with data. Whether it's building internal dashboards, deploying AI-powered tools, or creating self-service analytics platforms, Databricks Apps provide the foundation for scalable and secure data applications
Choosing the Right App Framework in Databricks: Flask, Dash, Streamlit & Gradio
When building apps in Databricks, developers have a variety of powerful Python frameworks at their disposal. These aren't new technologies - Flask, Dash, Streamlit, and Gradio have long been used to create interactive data and ML applications. What's new is how seamlessly they now run within Databricks, right next to your data and models.
But with so many options, the natural question is: When should you use which framework -and why?
This section breaks down the purpose, strengths, and ideal use cases of each framework so you can choose the one that best fits your workload.
Why These Frameworks?
Each of these tools was designed to solve a different problem:
- Flask gave Python developers a lightweight web server for building custom APIs and web apps.
- Dash emerged to simplify building analytical dashboards with rich interactivity.
- Streamlit aimed to let data scientists turn notebooks into UIs in minutes.
- Gradio lowered the barrier to showcasing ML models with real-time inputs and outputs.
Now that these are first-class citizens inside Databricks, you can run apps powered by them directly against live Delta tables, ML models, and streaming data.
Framework Breakdown
Flask -Build-Your-Own App, From Scratch
- Ideal For: Custom web apps, REST APIs, multi-page dashboards
- Strengths: Total flexibility and control, You define the routing, HTML structure, and logic, Can integrate ML models, render advanced UIs, or even embed dashboards
Use Flask if you're building a tailored experience or need full control over layout, access control, and user interaction.
Dash -Visual Analytics with Python & Plotly
- Ideal For: KPI dashboards, analytical apps, time series explorers
- Strengths: React-style interface built entirely in Python,Beautiful visualizations with Plotly baked in,Callback mechanism makes charts interactive and reactive.
Dash is great for showing metrics and trends -think of it as your real-time data observatory inside Databricks.
Streamlit -The Notebook-to-App Workflow
- Ideal For: Rapid ML demos, data profiling tools, form-based apps
- Strengths: Extremely fast to build with minimal code,Built-in widgets like sliders, dropdowns, and file uploaders,Easy integration with charts (Plotly, Matplotlib, Altair, etc.)
Streamlit is perfect for data scientists who want to move fast -just add a few st.sidebar or st.metric calls, and you're ready to go live.
Gradio -Instant ML Model Interfaces
- Ideal For: NLP/CV model demos, real-time predictions, user feedback collection
- Strengths: Drag-and-drop style UI for models, supports text, images, audio, and video inputs,Minimal setup to expose your model as a web app.
Gradio shines when you want to demo an ML model's power -just wrap your function and expose it with a UI.
Summary Comparison
Putting It All Together: A Complete Databricks App in Action
Before we dive into the file structure and deployment steps, let's quickly revisit what this project is all about.
We're building a Customer Sentiment Dashboard that reads feedback from a Delta table (customer_feedback) and visualizes it using an interactive, visually rich UI built with Flask. The dashboard allows users to:
- Filter feedback by region
- View KPIs like positive, neutral, and negative sentiment counts
- Explore sentiment score distribution and breakdown via vibrant visuals
- Read the top 5 positive and negative feedback comments
- Enjoy a sleek UI with custom color palettes and fonts
The app runs entirely within Databricks Apps, using Python, Plotly, and HTML templating -all packaged as a deployable app with a few essential files.
Let's take a quick look at the core components that power a Databricks App. These are the building blocks that define the app's behavior, dependencies, and interface.
1. app.py -Your Main Application Logic
This is the heart of your app.
- It defines the UI using a Python framework like Flask, Dash, Streamlit, or Gradio
- Handles routing, data queries, visuals, and user interactions
- Connects directly to your Delta tables using the Databricks SQL connector
- Can render HTML templates, serve dynamic charts, and expose ML model results
from flask import Flask, render_template_string, request
import pandas as pd
import plotly.express as px
from databricks import sql
app = Flask(_name_)
# Set up connection
connection = sql.connect(
server_hostname="adb-8333330282xxxxxx.13.azuredatabricks.net",
http_path="/sql/1.0/warehouses/a54c65xxxxxxdb53",
access_token="dapi3d2be2a3a92e29xxxxxxb12916727bde-3"
)
@app.route('/', methods=["GET"])
def index():
selected_region = request.args.get("region")
# Load data
query = "SELECT region, sentiment_score, sentiment_label, feedback_text FROM unitygo.default.customer_feedback"
df = pd.read_sql(query, connection)
# Filter region
region_options = sorted(df["region"].dropna().unique())
if selected_region:
df = df[df["region"] == selected_region]
# KPI Metrics
total_count = len(df)
# Clean up sentiment labels
df["sentiment_label"] = df["sentiment_label"].str.strip().str.lower()
# KPI Metrics (after cleanup)
positive_count = len(df[df["sentiment_label"] == "positive"])
neutral_count = len(df[df["sentiment_label"] == "neutral"])
negative_count = len(df[df["sentiment_label"] == "negative"])
# Histogram
hist_fig = px.histogram(
df, x="sentiment_score", color="sentiment_label", nbins=20,
title="Sentiment Score Distribution",
color_discrete_map={"positive": "#2ecc71", "neutral": "#f1c40f", "negative": "#e74c3c"},
template="plotly_dark"
)
hist_html = hist_fig.to_html(full_html=False)
# Pie chart
pie_fig = px.pie(df, names="sentiment_label", title="Sentiment Breakdown",
color_discrete_map={"positive": "#2ecc71", "neutral": "#f1c40f", "negative": "#e74c3c"},
hole=0.4)
pie_html = pie_fig.to_html(full_html=False)
# Feedback highlights
top_positive = df[df["sentiment_label"] == "positive"].sort_values(by="sentiment_score", ascending=False).head(5)
top_negative = df[df["sentiment_label"] == "negative"].sort_values(by="sentiment_score").head(5)
print("Sample feedback data:\n", df.head())
print("Unique sentiment labels after cleanup:", df["sentiment_label"].unique())
return render_template_string("""
<html>
<head>
<title>Customer Feedback Dashboard</title>
<style>
body {
background: linear-gradient(135deg, #f0f4ff, #ffffff);
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
padding: 20px;
color: #333;
}
h1 {
text-align: center;
font-size: 3em;
color: #1e3a8a;
margin-bottom: 20px;
}
h2, h3 {
color: #1f2937;
}
form {
margin-bottom: 30px;
text-align: center;
}
select {
font-size: 1.1em;
padding: 8px 12px;
border-radius: 6px;
border: 1px solid #ccc;
}
.kpi-container {
display: flex;
justify-content: center;
gap: 30px;
margin-bottom: 30px;
}
.kpi-card {
background-color: #ffffff;
border-radius: 16px;
padding: 20px 30px;
box-shadow: 0 6px 16px rgba(0, 0, 0, 0.1);
text-align: center;
flex: 1;
max-width: 200px;
}
.kpi-title {
font-size: 1.2em;
color: #6b7280;
margin-bottom: 10px;
}
.kpi-value {
font-size: 2.2em;
font-weight: bold;
color: #1d4ed8;
}
.plot-container {
background-color: #ffffff;
border-radius: 16px;
padding: 25px;
margin-bottom: 30px;
box-shadow: 0 6px 20px rgba(0, 0, 0, 0.08);
}
ul {
list-style-type: disc;
padding-left: 25px;
}
li {
margin-bottom: 8px;
}
</style>
</head>
<body>
<h1>Customer Feedback Dashboard</h1>
<form method="GET" style="text-align:center;">
<label for="region">Filter by Region:</label>
<select name="region" onchange="this.form.submit()">
<option value="">All</option>
{% for region in regions %}
<option value="{{ region }}" {% if selected_region == region %}selected{% endif %}>{{ region }}</option>
{% endfor %}
</select>
</form>
<div class="kpi-container">
<div class="kpi-card">
<h3>Total Feedback</h3>
<p>{{ total }}</p>
</div>
<div class="kpi-card">
<h3>Positive</h3>
<p>{{ positive }}</p>
</div>
<div class="kpi-card">
<h3>Neutral</h3>
<p>{{ neutral }}</p>
</div>
<div class="kpi-card">
<h3>Negative</h3>
<p>{{ negative }}</p>
</div>
</div>
<h2> Sentiment Score Histogram</h2>
{{ hist_plot | safe }}
<h2> Sentiment Distribution</h2>
{{ pie_plot | safe }}
<h2> Feedback Highlights</h2>
<div class="feedback-section">
<div class="feedback-box">
<h3>Top Positive Feedback</h3>
<ul>
{% for feedback in top_pos %}
<li>{{ feedback }}</li>
{% endfor %}
</ul>
</div>
<div class="feedback-box">
<h3>Top Negative Feedback</h3>
<ul>
{% for feedback in top_neg %}
<li>{{ feedback }}</li>
{% endfor %}
</ul>
</div>
</div>
</body>
</html>
""",
total=total_count,
positive=positive_count,
neutral=neutral_count,
negative=negative_count,
hist_plot=hist_html,
pie_plot=pie_html,
top_pos=top_positive["feedback_text"].tolist(),
top_neg=top_negative["feedback_text"].tolist(),
regions=region_options,
selected_region=selected_region)
if _name_ == "_main_":
app.run(host='0.0.0.0')
You can also use app.py to register MLflow models or pull secrets from Unity Catalog if needed.
2. requirements.txt -Python Dependencies
This file lists the external Python libraries your app needs.
flask
gunicorn
plotly
pandas
sqlalchemy
databricks-sql-connector
When the Databricks App is deployed, these packages are automatically installed in a virtual environment isolated to your app -no need to worry about conflicts with notebook environments.
3. app.yaml -Metadata & Configuration
This YAML file tells Databricks how to run and describe your app.
Typical fields include:
command: ["gunicorn", "-b", "0.0.0.0", "app:app"]
You can also add optional config like:
- environment: to define environment variables
- description: for App Catalog listing
- permissions: to set who can run it
This file is required for deployment -it's how Databricks knows what to do with your code.
How to Create and Launch a Databricks App
Here's how you can create and deploy a custom Databricks App from your own code:
Step-by-Step App Creation in Databricks
- Navigate to Your Workspace
Go to the folder where you want to create the app. - Click on "+ Create" (Top Right)
From the menu, select App.
- Choose a Template
You'll be prompted to pick an app template.
Select "Custom" to build an app from your own files.
- Fill in the App Name and Description
Enter a name (required) and an optional description for your app. - Click "Create"
This creates the app entity in Databricks. - Click "Deploy App" (Top Right of App View)
Once inside the app screen, click "Deploy App" to set it up with your files. - Select Folder Location
In the deployment dialog, choose the folder containing your: - app.py
- app.yaml
- requirements.txt
(All must be in the same directory.) - Click "Deploy"
Databricks will install dependencies, spin up the environment, and start the app.
Click "Open App"
Once the app is live, copy the app URL and paste it in a browser to interact with it in the Databricks UI.
- You can review your deployed apps from the Apps Tab of Compute section:
Final App Preview: What the UI Looks Like
Once you've deployed your Databricks App, this is what the User Interface (UI) will look like. It's an interactive dashboard for customer feedback visualization that includes dynamic charts, KPI cards, and feedback highlights.
Key Features of the UI:
- Interactive Charts: Users can interact with sentiment distributions and histograms.
- Feedback Highlights: The UI dynamically lists top positive and negative feedback based on the chosen region or global selection.
- KPIs: Real-time sentiment KPIs are displayed to help stakeholders get quick insights.
Here's how the UI looks:
- Sentiment Score Histogram
A beautiful histogram with color-coded sentiment scores (positive, negative, neutral) is shown. The users can interact with it by selecting different regions, which will update the data visualized. - Sentiment Breakdown Pie Chart
A pie chart gives a breakdown of how positive, neutral, and negative feedback is distributed, all displayed with vibrant colors for better clarity. - Dynamic Feedback Table
A table lists the most relevant feedback (both positive and negative), and it's fully interactive. The feedback changes based on the region selected.
Conclusion: Unlocking the Power of Databricks Apps
In this blog, we've explored how Databricks Apps can revolutionize how we build and deploy interactive, data-driven applications. We've demonstrated how to create a customer feedback dashboard using Flask within the Databricks environment, integrating data visualizations like sentiment histograms, pie charts, and real-time feedback KPIs. With just a few lines of code, we built a powerful tool that turns raw data into actionable insights.
Whether you're a data scientist, engineer, or business analyst, Databricks Apps opens up a world of possibilities. The ability to easily create and deploy custom applications with integrated data processing and visualization allows teams to make faster, data-backed decisions.
Key Takeaways:
- Databricks Apps allows for seamless integration of Python libraries like Flask and Plotly for building interactive dashboards.
- The simplicity of deploying these apps within the Databricks platform means you can focus more on your code and less on infrastructure.
- The potential for creating custom, data-driven applications with interactive visuals is limitless, offering significant improvements in data analysis and reporting.
As organizations move towards data-driven decision-making, the ability to turn data into interactive insights has never been more critical. Databricks Apps empowers you to take your analytics to the next level, transforming raw data into engaging, real-time applications for better business outcomes.
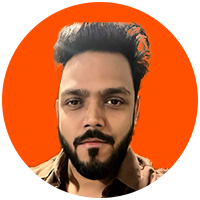
AUTHOR - FOLLOW
Sumer Anand
Manager, Data Engineering
Next Topic
Architecting AI Agents with Databricks: From Vector Search to Foundation Models
Next Topic